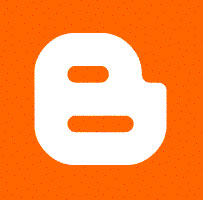
Ajax
LOG-IN SCRIPT FOR AJAX
Author: Ken V.
Language: Ajax
Database: Firebird Interbase
<?php
class Login{
private $dbInstance;
private $resultmessage;
private $isactive;
private $connected = false;
public function __construct(){
$this->resultmessage['success'] = false;
DBInstance::setDBEngine(DBASE_ENGINE);
DBInstance::setDBase(ECLRDB);
$this->dbInstance = DBInstance::getInstance();
if(!$this->dbInstance){
$this->resultmessage['errors']['reason'] = DBInstance::getDbError();
}else{
$this->connected = true;
}
if(!session_start()){
session_start();
}
}
public function getIsactive_0($userid){
$output = array();
if($this->connected){
$trans = ibase_trans(IBASE_DEFAULT,$this->dbInstance);
try{
$query = "select * from users where
userid='{$userid}'";
$pstmt = ibase_prepare($trans,$query);
$result = ibase_execute($pstmt);
if(!$result){
throw new RuntimeException(ibase_errmsg(), ibase_errcode());
}
while($row=ibase_fetch_object($result)){
$output[] = array(
$this->isactive = trim($row->active),
);
}
ibase_commit($trans);
}catch(Exception $e){
ibase_rollback($trans);
}
}
return $output;
}
public function doLogin(){
if($this->connected){
$active = $this->getIsactive_0($_REQUEST['u']);
$username = $_REQUEST['u'];
$password = $_REQUEST['p'];
$query = "select * from users where userid=? and";
if($this->isactive == 0){
$query .="active='0'";
}else{
$query .="active='1'";
}
try{
$transaction = ibase_trans(IBASE_DEFAULT,$this->dbInstance);
$pstmt = ibase_prepare($transaction,$query);
$result = ibase_execute($pstmt, $username);
if(!$result){
throw new RuntimeException(ibase_errmsg(),ibase_errcode());
}
$row = ibase_fetch_object($result);
if(!$row){
$query = "select * from users where";
if($this->active== 0){
$query .="active='0'";
}else{
$query .="active='1'";
}
$pstmt = ibase_prepare($transaction,$query);
$result = ibase_execute($pstmt,$username);
if(!$result){
throw new RuntimeException(ibase_errmsg(), ibase_errcode());
}
$row=ibase_fetch_object($result);
if(!$row){
$this->resultmessage['errors']['reason'] = 'Invalid login. Please try again.';
}else{
if($row->password!= $password){
$this->resultmessage['errors']['reason'] = 'Invalid password. Please try again.';
}else{
if($this->isactive != 1){
$this->resultmessage['errors']['reason']='You have already created your Account Thank you.';
}else{
$this->resultmessage['success'] = true;
$_SESSION['roleidtims'] = '0';
$_SESSION['accountname'] = trim($row->userid);
$this->resultmessage['page']['redirect'] = 'index.php?page=buildui&action=buildUI';
}
}
}
}else{
if($row->USERPASSWD != $password){
$this->resultmessage['errors']['reason'] = 'Invalid password. Please try again.';
}else if($row->ACCTCREATED != 1){
$this->resultmessage['errors']['reason'] = 'Account not yet created. Please create an account to continue.';
}else if($row->ISACTIVE != 1){
$this->resultmessage['errors']['reason']='You have already created your Account.
Thank you.';
}else{
$_SESSION['accountname'] = trim($row->userid);
$_SESSION['fullname'] = $this->nameReformat($row->firstname. " " . $row->LASTNAME);
$_SESSION['isadmin']= trim($row->admin);
$_SESSION['positioncode']= trim($row->position);
$this->resultmessage['success'] = true;
//Redirect page to index.php after login fails.
$this->resultmessage['page']['redirect'] = 'index.php?page=buildui&action=buildUI';
}
}
if(!$result){
throw new RuntimeException(ibase_errmsg(),ibase_errcode());
}
$row = ibase_fetch_object($result);
}catch(Exception $e){
ibase_rollback($transaction);
$this->resultmessage['errors']['reason'] = $e->getMessage();
}
}
//echo $this->isactive;
echo json_encode($this->resultmessage);
}
public function nameReformat($name){
$tempArr = split(" ", $name);
$fname = "";
for($counter=0;$counter<count($tempArr);$counter++){
$fname .= ucfirst(strtolower($tempArr[$counter])) . ' ';
}
return trim($fname);
}
}
$login = new Login();
$login->doLogin();
?>
This code is Copyrighted by the owner.
Copyright © 2010-2011 Kevern Solutions